Python Libraries for Data Science: A Comprehensive Guide
Do you know that data science has become an essential field in the current digital era? It is transforming the way businesses make decisions and unlocking insights from massive datasets. Python, with its rich ecosystem of libraries, has emerged as a dominant force in data science.
In this blog, we will look into various Python libraries in data science that cater to different aspects of data science, are categorized for easy navigation, and will touch on statistical analysis and data preprocessing techniques.
What are Python Libraries?
Python libraries are like toolboxes filled with pre-made tools that you can use to make coding easier and faster. They come with ready-to-use functions for different jobs, such as analyzing data, training machines, and creating visuals. These libraries are like shortcuts that help you do more with Python, whether you’re working on data, machines, or other cool stuff. Learn data science to improve your proficiency in Python libraries.
Python Libraries in Data Manipulation and Analysis
There are several popular Python libraries in data science for data manipulation and analysis. Some of the most widely used ones include:
Pandas
- Pandas is the cornerstone library for data manipulation and analysis in Python. It provides data structures and functions for efficiently handling and processing structured data, making it a go-to tool for tasks like data cleaning, transformation, and aggregation.
Example
Here is an example using Pandas.
import pandas as pd data = {‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’, ‘David’], ‘Age’: [25, 30, 28, 22], ‘Salary’: [50000, 60000, 55000, 45000]} df = pd.DataFrame(data) young_employees = df[df[‘Age’] < 30] df[‘Bonus’] = df[‘Salary’] * 0.1 print(df) |
This example demonstrates creating a DataFrame, filtering data based on a condition, and adding a new column to the DataFrame using Pandas.
NumPy
- It is the foundation of numerical computing in Python. It offers powerful array operations and mathematical functions, enabling efficient handling of multi-dimensional arrays and matrices.
Example
Here is an example using NumPy.
import numpy as np a, b = np.array([1, 2, 3, 4, 5]), np.array([5, 4, 3, 2, 1])add, mult = a + b, a * bmean_a, dot = np.mean(a), np.dot(a, b) print(“a:”, a, “b:”, b, “Add:”, add, “Mult:”, mult, “Mean a:”, mean_a, “Dot:”, dot) |
This example demonstrates some of the basic operations you can perform using NumPy, such as element-wise addition and multiplication, calculating the mean of an array, and computing the dot product of two arrays.
Dask
- t is a powerful parallel computing library designed to handle datasets that exceed memory limits. With its dynamic task scheduling and parallel execution capabilities, Dask empowers data scientists to efficiently process and analyze vast amounts of data, unlocking new possibilities for advanced data-driven insights.
Example
Here is an example using Dask.
import dask.array as da x = da.random.random((100000, 100000), chunks=(1000, 1000))result = (x + x.T).compute() print(result) |
This code snippet uses Dask to create a distributed array with random data, breaks it into smaller chunks, and performs parallel element-wise addition with its transpose. The final result is computed using dynamic task scheduling and stored in memory before being printed.
Get an Assured Job guarantee by enrolling in our data science placement guarantee course.
Python Libraries in Visualization
Python libraries offer a rich ecosystem for data visualization. These libraries provide a wide range of options, from basic charts to complex interactive visualizations. Let’s explore some popular Python libraries for data visualization:
Matplotlib
Matplotlib is a versatile plotting library that offers a wide range of static, interactive, and 3D visualization options. It’s highly customizable and widely used for creating publication-quality plots.
Example
Here is an example using Matplotlib.
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5]y = [2, 4, 6, 8, 10] plt.plot(x, y, label=’Line Plot’)plt.xlabel(‘X-axis’)plt.ylabel(‘Y-axis’)plt.title(‘Simple Line Plot’)plt.legend()plt.show() |
This code will generate a basic line plot with labeled axes and a legend using Matplotlib. You can customize the plot further by adjusting various parameters and adding more data and plot types.
Seaborn
- Seaborn builds on top of matplotlib and provides a high-level interface for creating attractive and informative statistical visualizations. It simplifies the creation of complex visualizations such as heat maps and Violin plots.
Example
Here is an example using Seaborn.
import seaborn as snsimport numpy as npimport matplotlib.pyplot as plt data = np.random.rand(5, 5)sns.heatmap(data, annot=True, cmap=’viridis’)plt.title()plt.show() |
This code uses Seaborn and NumPy to create a heatmap. Random data forms a 2D array, and Seaborn’s heatmap function visualizes it. Annotations and a color map are added with annot=True and cmap. plt.title gives a title, and plt.show() displays the plot.
Plotly
- Plotly is a popular library for creating interactive visualizations and dashboards. It supports a variety of chart types and can be embedded in web applications for dynamic data exploration.
Example
Here is an example using Plotly.
import plotly.express as px data = {‘Category’: [‘A’, ‘B’, ‘C’, ‘D’], ‘Value’: [25, 40, 10, 30]}fig = px.bar(data, x=’Category’, y=’Value’, title=’Interactive Bar Chart’)fig.show() |
This example uses Plotly Express, which is a higher-level interface for creating various types of visualizations with minimal code. You can explore more chart types and customization options in the Plotly documentation.
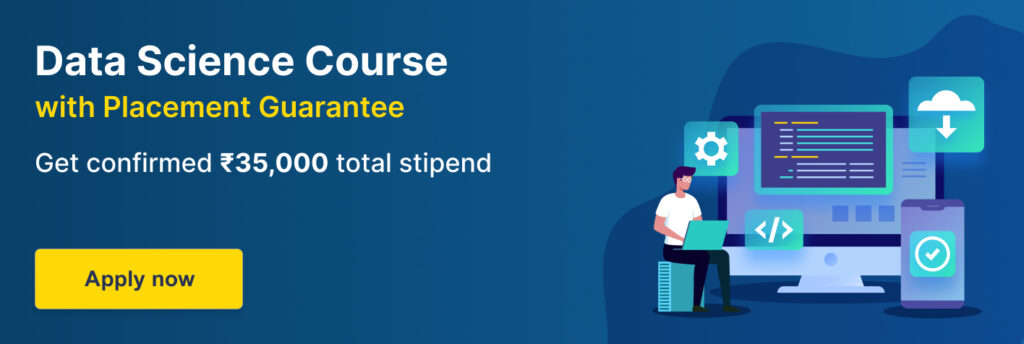
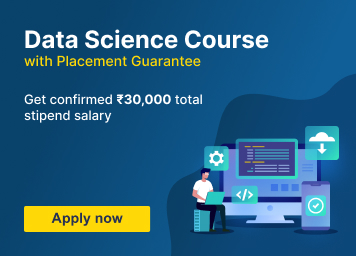
Python Libraries in Machine Learning
Python libraries in data science have emerged as a dominant library in the field of machine learning, these libraries provide powerful tools for various tasks let’s look at some of the important machine learning libraries:
Scikit-Learn
- This is a comprehensive machine-learning library that offers a wide range of algorithms for classification, regression, clustering, and more. It provides a consistent API for model training, evaluation, and deployment.
Example
Here is an example using Scikit-Learn.
from sklearn.datasets import load_irisfrom sklearn.model_selection import train_test_splitfrom sklearn.neighbors import KNeighborsClassifierfrom sklearn.metrics import accuracy_score X, y = load_iris(return_X_y=True)X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) model = KNeighborsClassifier(n_neighbors=3).fit(X_train, y_train)y_pred = model.predict(X_test) accuracy = accuracy_score(y_test, y_pred)print(f”Accuracy: {accuracy:.2f}”) |
In this example, we load the Iris dataset, split it into training and testing sets, initialize a KNeighborsClassifier model, train it on the training data, make predictions on the test data, and calculate the accuracy of the model’s predictions.
TensorFlow and Keras
- TensorFlow is an open-source machine learning framework that excels at deep learning tasks. Keras, which is now part of TensorFlow, provides a high-level interface for building and training neural networks.
Example
Here is an example using TensorFlow and Keras.
import tensorflow as tffrom tensorflow.keras.models import Sequentialfrom tensorflow.keras.layers import Flatten, Dense (x_train, y_train), (x_test, y_test) = tf.keras.datasets.fashion_mnist.load_data()x_train, x_test = x_train / 255.0, x_test / 255.0 model = Sequential([Flatten(input_shape=(28, 28)), Dense(128, activation=’relu’), Dense(10, activation=’softmax’)])model.compile(optimizer=’adam’, loss=’sparse_categorical_crossentropy’, metrics=[‘accuracy’])model.fit(x_train, y_train, epochs=5)test_loss, test_acc = model.evaluate(x_test, y_test)print(f”Test accuracy: {test_acc}”) |
This example involves importing TensorFlow and Keras, loading/preprocessing Fashion MNIST data, creating a simple neural network, compiling it with an optimizer/loss, training on training data, and evaluating on test data.
PyTorch
- PyTorch is another powerful deep learning framework that offers dynamic computation graphs and a more intuitive programming interface. It’s favored by researchers and developers for its flexibility and ease of use.
Example
Here is an example using PyTorch.
import torchimport torch.nn as nnimport torch.optim as optim class SimpleNN(nn.Module): def __init__(self): super(SimpleNN, self).__init__() self.fc1 = nn.Linear(784, 128) self.fc2 = nn.Linear(128, 64) self.fc3 = nn.Linear(64, 10) def forward(self, x): return self.fc3(torch.relu(self.fc2(torch.relu(self.fc1(x))))) model = SimpleNN()criterion = nn.CrossEntropyLoss()optimizer = optim.SGD(model.parameters(), lr=0.01) sample_input = torch.randn(64, 784)outputs = model(sample_input)loss = criterion(outputs, torch.randint(10, (64,))) optimizer.zero_grad()loss.backward()optimizer.step() |
This example demonstrates a basic neural network architecture and training loop using PyTorch. You can customize and extend this example to suit your specific needs and datasets.
Python Libraries in Data Access and Storage
Python libraries in data science offer a rich ecosystem for data access and storage, empowering developers to efficiently manage and manipulate data. Let’s look at tools for connecting to databases and performing other tasks:
SQLAlchemy
SQLAlchemy is a SQL toolkit and Object-Relational Mapping (ORM) library. It provides a powerful way to interact with databases using Python, enabling seamless integration of data storage and analysis.
Example
Here is an example using SQLAlchemy.
from sqlalchemy import create_engine, Column, Integer, Stringfrom sqlalchemy.orm import sessionmakerfrom sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = ‘users’ id = Column(Integer, primary_key=True) name = Column(String) age = Column(Integer) engine = create_engine(‘sqlite:///example.db’, echo=True)Session = sessionmaker(bind=engine)session = Session() Base.metadata.create_all(engine)session.add(User(name=’John Doe’, age=30))session.commit() user_query = session.query(User).filter_by(name=’John Doe’).first()print(user_query.name, user_query.age) user_query.age = 31session.commit() session.delete(user_query)session.commit() session.close() |
This example demonstrates how to define a User data model, create an SQLite database, perform CRUD (Create, Read, Update, Delete) operations, and close the database session using SQLAlchemy.
Apache Spark
Although primarily written in Scala, Apache Spark provides a Python API (PySpark) for distributed data processing. It’s designed for handling large-scale data processing tasks efficiently.
Example
Here is an example using Apache Spark.
from pyspark import SparkContext, SparkConf conf = SparkConf().setAppName(“WordCount”).setMaster(“local”)sc = SparkContext(conf=conf) text_file = sc.textFile(“path/to/your/textfile.txt”)word_counts = text_file.flatMap(lambda line: line.split(” “)).map(lambda word: (word, 1)).reduceByKey(lambda a, b: a + b) for (word, count) in word_counts.collect(): print(f”{word}: {count}”) sc.stop() |
Make sure to replace “path/to/your/textfile.txt” with the actual path to your text file. This example reads a text file, splits it into words, counts the occurrences of each word, and prints the word counts.
Python Libraries in Natural Language Processing (NLP)
Natural Language Processing (NLP) involves the interaction between computers and human language. Python libraries in data science have become the go-to language for NLP tasks due to their rich ecosystem. Here are some common Python libraries widely used in NLP:
NLTK (Natural Language Toolkit)
NLTK is a comprehensive library for NLP and text analysis tasks. It offers a wide range of tools for stemming, tokenization, parsing, and sentiment analysis.
Example
Here is an example using the NLTK library.
import nltknltk.download(‘punkt’)from nltk.tokenize import word_tokenize sentence = “NLTK is a powerful library for natural language processing.”tokens = word_tokenize(sentence)print(tokens) |
This code imports NLTK, downloads the required tokenizer resource, tokenizes the given sentence into individual words, and then prints the resulting tokens. Make sure you have NLTK installed (pip install nltk) and the necessary resources downloaded (nltk.download(‘punkt’)) before running the code.
spaCy
spaCy is a fast and efficient NLP library that focuses on production use cases. It provides pre-trained models and supports various languages for tasks like part-of-speech tagging and named entity recognition.
Example
Here is an example using the spaCy library.
import spacy nlp = spacy.load(“en_core_web_sm”)text = “Apple is looking at buying U.K. startup for $1 billion.”doc = nlp(text) for token in doc: print(f”Token: {token.text}\tPOS: {token.pos_}\tNER: {token.ent_type_}”) |
Remember to install spaCy and the en_core_web_sm model as mentioned earlier. This code will output a list of tuples containing each token and its corresponding part-of-speech tag.
Statistical Analysis in Python Libraries
Statistical analysis involves techniques for summarizing, interpreting, and drawing conclusions from data. It encompasses tasks such as hypothesis testing, probability distributions, and descriptive statistics. Python offers powerful libraries that streamline the following processes:
Probability Distributions
Python’s scipy.stats module provides a comprehensive suite of probability distributions. You can generate random samples, calculate probability density functions (PDFs), cumulative distribution functions (CDFs), and more.
Hypothesis Testing
Hypothesis testing is a way for us to make inferences about population parameters based on sample data. There are tools like scipy.stats and stats models that help us with this kind of testing. They make it easy to compare things like averages, proportions, and variations, and also to perform tests like t-tests, chi-squared tests, ANOVA, and regression analysis.
Data Preprocessing in Python Libraries
Data preprocessing is a foundational step in data science libraries in Python. The workflow of Python involves cleaning, transforming, and organizing raw data to make it suitable for analysis and modeling. Inaccurate or incomplete data can lead to erroneous insights and models, making data preprocessing an essential process. Let’s look at key aspects of data preprocessing:
Handling Missing Values
They are a common occurrence in datasets and can adversely affect the quality of analysis. Python’s panda library provides efficient methods to handle missing data. These methods enable you to identify, impute, or remove missing values as needed.
Data Transformation
It is the process of converting data into a format suitable for analysis. This may involve scaling numerical features, encoding categorical variables, and ensuring data uniformity. Libraries like scikit-learn offer functions that standardize numerical features and encode categorical variables.
Dealing with Outliers
Outliers are data points that deviate significantly from the majority of the data. They can distort statistical analyses and machine learning models. Identifying and addressing outliers is crucial. Python libraries like scipy.stats and seaborn provide statistical methods and visualizations to detect and handle outliers.
Data Normalization
This involves scaling features to a common scale to ensure fair comparisons. Scaling is particularly important for algorithms that are sensitive to feature scales, such as gradient-based optimization algorithms. Libraries like scikit-learn offer various normalization techniques, including Min-Max scaling and Z-score normalization.
Data Aggregation and Transformation
Data often needs to be aggregated or transformed to extract meaningful insights. Python’s Panda library provides powerful tools for data aggregation, including the ability to group data by specific attributes. Pivot tables are another effective way to transform and summarize data.
Conclusion
Python libraries in data science play a pivotal role in making data science accessible and efficient. Whether you’re cleaning and analyzing data, creating captivating visualizations, or building intricate machine-learning models, there’s a Python library tailored to your needs. These Python libraries will serve as a starting point for your data science journey, enabling you to unlock valuable insights from data and drive informed decisions.